Code formatting and comments are one of those things that you only notice once they are absent. If used to properly display and document code, you only notice their existence the first time you are confronted with a block of code where they are missing.
Most people who run or own a website sooner or later get confronted with making changes to the code base. This can be when creating a new HTML element, inserting some custom CSS or making changes to a WordPress PHP template.
To make sure it doesn’t end in a mess, in this post we will look at best practices for code formatting and comments. You will learn why they are essential and also how to use them properly.
Since there are many programming and markup languages out there with different conventions, we will limit ourselves to the most common in web design, meaning HTML, CSS, PHP, and JavaScript.
Why Should You Format and Document Your Code?
Before getting into the how-to, let’s first talk about why this even matters.
Code Formatting is Not Necessary for Functionality
First of all, formatting and commenting code is not actually needed for a function to work. Machines such as browsers are perfectly capable of reading and executing it without.
It’s why you can use techniques like minification to speed up your WordPress site.
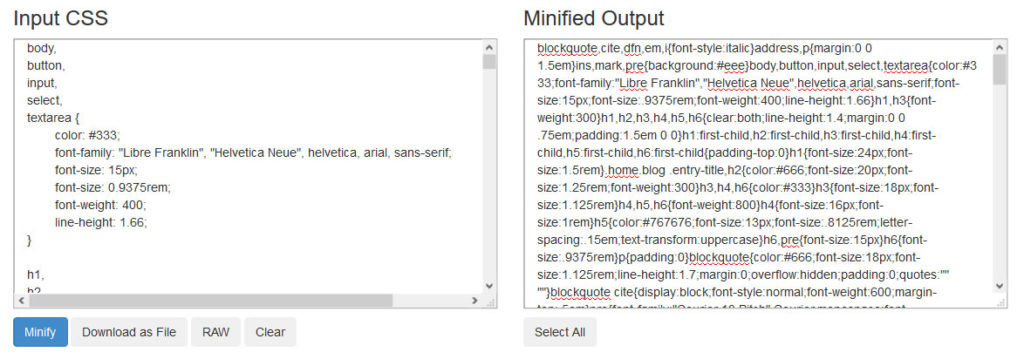
A computer doesn’t care if the code looks pretty as long as it’s correct and doesn’t produce errors.
However, It Makes Markup More Humanly Readable
Yet, you know who does care about code formatting and comments? Humans. People like you and me who need to read, understand, and modify code from time to time.
In those instances, it’s much easier if you have proper formatting and/or comments that make the code easier to understand. Not using formatting in code is equivalent to not using formatting in writing. You wouldn’t read this post either if I did away with all the paragraphs and headings, no matter how good the content is otherwise.
For that reason, people have come up with conventions to make sure code is as understandable as possible. This helps avoid errors, troubleshoot problems and makes maintaining someone else’s (or even your own) work much easier.
Essential Parts of Code Formatting
However, it’s important to notice that there is no one ultimate way of styling code.
For example, different formatting can make sense for different markup. Applying CSS formats to HTML or vice versa wouldn’t necessarily make either easier to understand.
Therefore, there are differing conventions depending on the language that you are using. Yet, all of them basically revolve around the following:
- Indentation
- White space
- Capitalization and naming conventions
- Style and spelling of functions, variables and more
- Use and style of comments
Within those broader conventions, developers often use their own style according to their preference. However, there are some elements of code formatting and commenting that are pretty universal and in the following, we will look at those.
How to Format Code – The Basics
From here on out, we will give you some guidelines on how to format code so that it’s easier to work with.
Layout – Indentation, Line Breaks, Formatting
Using proper layout helps identify parts of code that belong together, are dependent on one another, and more. The basic tools for that are indentation, line breaks, and other formatting options. Here’s how to use them in different programming languages.
HTML
In HTML markup, you usually indent elements to show that they are nested within one another.
You can either do so with a tab or several spaces. There are discussions about what is the right way to do so (surprise!) but you can go with what works for you.
Proper indenting also puts closing brackets of containers such as div elements at the same vertical level so it’s easy to see whether you have closed them or not. Adding comments (more on that below) makes that even clearer.
This is sometimes amplified by carriage returns that introduce even more space around elements.
CSS
Here’s some well-formatted CSS code:
.search-submit { border-radius: 0 2px 2px 0; bottom: 0; overflow: hidden; padding: 0; position: absolute; right: 0; top: 0; width: 42px; }
Why does it look good?
Many reasons: There’s space between operators, braces, and properties, the rules have been indented, are in alphabetical order and each has their own line and the whole thing is closed on an extra line.
An additional way to make CSS more understandable is to use shorthand. Compare these two snippets:
.footer { margin-bottom: 10px; margin-left: 5px; margin-right: 5px; margin-top: 10px; } .footer { margin: 10px 5px; }
They do the same but one is much shorter.
Finally, when you use media queries, make sure to nest them inside each other and indent them properly.
@media screen and (min-width: 56.875em) { .site-header { padding-right: 4.5455%; padding-left: 4.5455%; } }
PHP
Similar rules apply to PHP code. Here’s an example from the Twenty Nineteen functions.php
file:
function twentynineteen_widgets_init() { register_sidebar( array( 'name' => __( 'Footer', 'twentynineteen' ), 'id' => 'sidebar-1', 'description' => __( 'Add widgets here to appear in your footer.', 'twentynineteen' ), 'before_widget' => '', 'before_title' => '', 'after_title' => '
', ) ); } add_action( 'widgets_init', 'twentynineteen_widgets_init' );
Notice the indents and line breaks here as well? Also, pay attention that all =>
have all been placed at the same height. This further increases readability.
JavaScript
Finally, here is an example from JavaScript:
const HeadingColorUI = memo( function( { textColorValue, setTextColor, } ) { return ( ); } );
You can see the same principles at work.
Naming Conventions and Capitalization
Another part of making code readable is to optimize its content. Here, it is important that what you write makes sense and people can understand what each element, function or variable does.
That way, they will get at a glance what they do and where to make changes. Here’s how to do that.
Be Descriptive
The first thing is that the names you use aren’t random but actually explain what a piece of code does.
unregister_sidebar( 'header-right' );
Anyone examining your work should be able to understand the function of each element immediately. Trust me, you will kick yourself in the future if you don’t adhere to this. There’s nothing worse than getting mad at your past self for being lazy.
Learn About Conventions
As mentioned earlier, different programming and markup languages have different conventions and it makes sense to adhere to them.
When it comes to naming, in HTML and CSS everything is usually lowercase. This includes element names, attributes, values, selectors, properties as well as HTML classes and ids.
However, as we have learned above, that’s not the case for every programming language. In PHP and JavaScript, you will also see things in camelCase
, under_scores
or with-hyphens
.
Which One is Correct?
Well, all of them work, so there is no incorrect solution (except hyphens in JavaScript, which can come off as subtractions, so don’t use them). Consequently, the most important thing here is that you decide on one and stick with it.
Aside from that, it makes sense to look into the convention of the platform you are working in. For example, WordPress encourages the use of underscores in PHP and camelCase in JavaScript.
So, if you are working with the WordPress platform, it’s a good idea to stick with these conventions, especially since many existing variables etc. are already in that format.
Code Comments Best Practices
With the above, chances are good that whenever another developer looks at your work, they will be able to understand what is going on quite quickly. In that case, your code is what people call “self-documenting”.
However, that is only the first step. In many cases, you need some actual documentation in the form of comments.
Learn How to Include Comments
Pretty much every programming language or markup allows you to input comments. That means you can mark text in a way so that browsers don’t execute it.
Here’s how to do that in the different languages:
- HTML — In HTML
. If it goes across several lines, you often put the opening and closing brackets on their own lines and place the indented comments in between.
- CSS — Anything in between
/*
and*/
is a comment. They can go across several lines. - PHP — PHP knows two types of comments: single line (marked with // or #) and multi-line (created with
/* ... */
, same as CSS). - JavaScript — Create a single-line comment with
//
. Multi-line works the same way as CSS/PHP.
A good example for code commenting is the WordPress child theme header.
/* Theme Name: Twenty Seventeen Theme URI: https://wordpress.org/themes/twentyseventeen/ Author: the WordPress team Author URI: https://wordpress.org/ Description: Twenty Seventeen brings your site to life with header video and immersive featured images. With a focus on business sites, it features multiple sections on the front page as well as widgets, navigation and social menus, a logo, and more. Personalize its asymmetrical grid with a custom color scheme and showcase your multimedia content with post formats. Our default theme for 2017 works great in many languages, for any abilities, and on any device. Version: 2.2 License: GNU General Public License v2 or later License URI: http://www.gnu.org/licenses/gpl-2.0.html Text Domain: twentyseventeen Tags: one-column, two-columns, right-sidebar, flexible-header, accessibility-ready, custom-colors, custom-header, custom-menu, custom-logo, editor-style, featured-images, footer-widgets, post-formats, rtl-language-support, sticky-post, theme-options, threaded-comments, translation-ready This theme, like WordPress, is licensed under the GPL. Use it to make something cool, have fun, and share what you've learned with others. */
Use Comments to Clarify Your Markup
However, what should you use this for?
Comments are aimed at helping anyone understand the code they are working with. This includes your future self (because you might not remember what you did earlier) or someone who takes over from you.
For that reason, it makes a lot of sense to leave information on what is going on. Describe what a particular function or code block does, which element a piece of CSS belongs to, etc.
//* Add new image sizes add_image_size( 'blog', 700, 300, TRUE );
Many developers also use them to create tables of content for their style sheets.
/*-------------------------------------------------------------- >>> TABLE OF CONTENTS: ---------------------------------------------------------------- 1.0 Normalize 2.0 Accessibility 3.0 Alignments 4.0 Clearings 5.0 Typography 6.0 Forms 7.0 Formatting 8.0 Lists 9.0 Tables 10.0 Links 11.0 Featured Image Hover 12.0 Navigation 13.0 Layout 13.1 Header 13.2 Front Page 13.3 Regular Content 13.4 Posts 13.5 Pages 13.6 Footer 14.0 Comments 15.0 Widgets 16.0 Media 16.1 Galleries 17.0 Customizer 18.0 SVGs Fallbacks 19.0 Media Queries 20.0 Print --------------------------------------------------------------*/
To be as helpful as possible, here too, make sure to be descriptive and clear in the content. Also, settle on one way of including comments and don’t deviate.
Code Formatting Tools
Remembering all the rules about proper formatting can be taxing. We are just covering the basics and already get a full article out of it. Therefore, to reduce the effort on your part, you can use a number of tools to make formatting more automatic and effortless.
Online Tools
Here are a number of tools which you can paste your code to and they will spit out a more beautiful version of it:
Code Editor Plugins
Aside from that, there are also plugins for code editors that can automatically do this kind of stuff.
- Atom Beautify
- HTML-CSS-JS Prettify for Sublime Text
- Prettier.io (works with Atom, Espresso, Sublime Text, WebStorm and more)
A quick Google search should give you options for your editor of choice. Code editors will also color elements differently, further increasing readability. You can already see this at work in the code examples in this post.
Style Guides
Finally, if you are mostly going the manual route, you can find a number of style guides out there to inspire you.
Code Formatting in a Nutshell
Code formatting and comments are useful tools to make any markup easier to understand, maintain, and troubleshoot. Above you have learned basic ways to use them to improve your workflow.
It bears repeating that none of the above is set in stone. While general conventions to make code look good exist, there are differences across languages, developers, etc.
The most important thing is that you stay consistent. Once you settle on a style of formatting, make sure not to deviate from it, especially within the same file or project. Otherwise, it will negate parts of why code formatting exists in the first place.
If you are working with a team, creating a style guide can help keep things consistent.
What are your favorite tools for code formatting and code comments? Anything to add to the above? Please let us know in the comments section below.
The post Code Formatting and Code Comments – A Beginner’s Guide to Do It Right appeared first on Torque.